What is JavaScript? What is Control Flow?
HTML structures the website and CSS beautifies it. JavaScript is a programming language, where it makes the website much more exciting and engaging.
All of HTML, CSS and Javascript has a "Control Flow." This is the order which the computer performs the written tasks in a sequential order.
Code is read from top to bottom, unless there are structures that changes the flow, e.g. a loops.
Control flow and loops are like a person's daily routine. This can be compared to driving a car.
A control flow is where a person puts on the seatbelt first before turning the car on, because of safety reasons and the rules we all have to follow. Always safety first!
In this process, a loop can be compared to different type of cars, e.g. electric and petrol cars.
With the petrol cars, you would put the key in and turn it to start the car up, however, with the electric cars, you would push a button and the car will automatically start.
With this example, it can be seen that everyone will have different control flows depending on what they prefer.
Loop is an option, which can speed up the process of control flow (electric cars starts on faster compared to petrol cars).
What is a DOM?
DOM stands for 'Document Object Model'. DOM is not a programming language, but acts as a binder to tie all of HTML, CSS and JavaScript together; enabling changes in the document structure, style and content. DOM consists of parents and children. It starts off with Window (Object), followed by Document (Object) then HTML. This shows that the Window is the root of hierarchy in the browser. Window allows the users to access the current URL, meaning that the user is able to get the information about the screen. The document object represent a read-only version of the HTML document, but it can also be modified if the user wishes to modify it. All of the alterations that is made on DOM through JavaScript represents what will be seen on browser. The DOM shows documents as nodes, this allows the programming languages to interact with the page.
Difference between accessing data from Arrays and Objects
To access data in arrays, the user would use the index numbers. The indexes in programs always start with 0 not 1, e.g. arr = array[1, 2, 3, 4] and calling arr[1] would show 2. There are three ways to access data in objects. const name = { girl: 'Sarah' };
- Dot property accessor, e.g. console.log(name.girl) would show Sarah
- Square brackets property accessor, e.g. console.log(name['girl']) would show Sarah
- Object destructuring, e.g. const {girl} = name; console.log(girl) would show Sarah
What are Functions?
Functions are expressions that are used in programming. This is very helpful because once a functions is written, it can be used multiple times in the program. This can save a lot of time as we wouldn't have to write same codes over and over again, but rather just use the function.
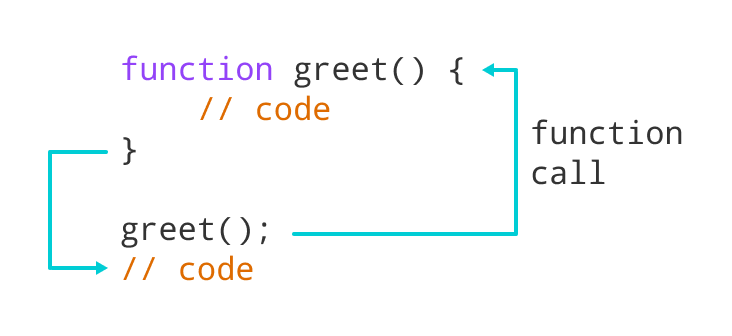